方針
・開発サーバーを起動中に、ミスがあればeslintエラーを表示してくれる。
・eslint-plugin-prettierは現在非推奨のため不使用。
手順
viteでReact(typescript)プロジェクトを作成する
yarn create vite my-app --template react-ts
上記のコマンドでviteが搭載されたreactアプリのテンプレートを一発で作成できます。
eslintの設定
eslintのインストールと初期設定
yarn add -D eslint
上記のコマンドでeslintのインストールを行います。
yarn eslint --init
上記のコマンドでeslintの初期設定を行います。
上記のコマンドでeslintの初期設定を行います。








いろいろ聞かれるので今回は上記の様に回答します。
{
"env": {
"browser": true,
"es2021": true
},
"extends": [
"eslint:recommended",
"plugin:react/recommended",
"plugin:@typescript-eslint/recommended",
+ "prettier"
],
"overrides": [],
"parser": "@typescript-eslint/parser",
"parserOptions": {
"ecmaVersion": "latest",
"sourceType": "module"
},
"plugins": [
"react",
"@typescript-eslint"
],
"rules": {
+ "react/react-in-jsx-scope": "off"
},
+ "settings": {
+ "react": {
+ "version": "detect"
+ }
+ }
}
ルート直下にある「/.eslintrc.json」を上記の様に修正します。
これがないと不要なエラーが出てしまいます。
eslintのコマンドを追加
{
"name": "my-app",
"private": true,
"version": "0.0.0",
"type": "module",
"scripts": {
"dev": "vite",
"build": "tsc && vite build",
"preview": "vite preview",
+ "lint": "eslint --fix --ext .tsx,.ts,.jsx,.js ."
},
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0"
},
"devDependencies": {
"@types/react": "^18.0.27",
"@types/react-dom": "^18.0.10",
"@typescript-eslint/eslint-plugin": "^5.55.0",
"@typescript-eslint/parser": "^5.55.0",
"@vitejs/plugin-react": "^3.1.0",
"eslint": "^8.36.0",
"eslint-plugin-react": "^7.32.2",
"typescript": "^4.9.3",
"vite": "^4.1.0"
}
}
上記のようにルート直下にある「/package.json」を修正し、コマンドを追加してください。
viteでeslintが起動する様に設定する
yarn add -D vite-plugin-checker
上記のコマンドでvite上でeslintを使う時に必要なライブラリをインストールします。
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
+ import checker from "vite-plugin-checker";
// https://vitejs.dev/config/
export default defineConfig({
plugins: [
react(),
+ checker({
+ typescript: false,
+ eslint: {
+ lintCommand: 'eslint --ext .tsx,.ts,.jsx,.js .',
+ },
+ }),
],
})
上記の様にルート直下にある「vite.config.js」を修正します。
prettierの設定
prettierのインストール
yarn add -D prettier
上記のコマンドでprettierをインストールしましょう。
prettierのコマンドの追加
{
"name": "my-app",
"private": true,
"version": "0.0.0",
"type": "module",
"scripts": {
"dev": "vite",
"build": "tsc && vite build",
"preview": "vite preview",
"lint": "eslint --fix --ext .tsx,.ts,.jsx,.js .",
+ "format": "prettier --write src"
},
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0"
},
"devDependencies": {
"@types/react": "^18.0.27",
"@types/react-dom": "^18.0.10",
"@typescript-eslint/eslint-plugin": "^5.55.0",
"@typescript-eslint/parser": "^5.55.0",
"@vitejs/plugin-react": "^3.1.0",
"eslint": "^8.36.0",
"eslint-plugin-react": "^7.32.2",
"prettier": "^2.8.4",
"typescript": "^4.9.3",
"vite": "^4.1.0",
"vite-plugin-checker": "^0.5.6"
}
}
上記のようにルート直下にある「/package.json」を修正し、コマンドを追加してください。
prettierの設定の追加
ルート直下に「.prettierrc」というファイルを作成し、以下のように修正しましょう。
{
"singleQuote": false
}
これで「シングルクオートではなくダブルクオートを使う」という設定がされました。
eslintとprettierを連携させるライブラリの設定
eslint-config-prettierのインストール
yarn add -D eslint-config-prettier
上記のコマンドでeslintとprettierを連携させるライブラリをインストールしましょう。
コマンドの追加
{
"name": "my-app",
"private": true,
"version": "0.0.0",
"type": "module",
"scripts": {
"dev": "vite",
"build": "tsc && vite build",
"preview": "vite preview",
"lint": "eslint --fix --ext .tsx,.ts,.jsx,.js .",
"format": "prettier --write src",
+ "conflict": "eslint-config-prettier 'src/**/*.{ts,tsx,js,jsx}'"
},
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0"
},
"devDependencies": {
"@types/react": "^18.0.27",
"@types/react-dom": "^18.0.10",
"@typescript-eslint/eslint-plugin": "^5.55.0",
"@typescript-eslint/parser": "^5.55.0",
"@vitejs/plugin-react": "^3.1.0",
"eslint": "^8.36.0",
"eslint-config-prettier": "^8.7.0",
"eslint-plugin-react": "^7.32.2",
"prettier": "^2.8.4",
"typescript": "^4.9.3",
"vite": "^4.1.0",
"vite-plugin-checker": "^0.5.6"
}
}
「yarn conflict」でeslintとprettierの設定に重複(衝突)するものはないかを確認してくれます。
全てを実行するコマンドの追加
{
"name": "my-app",
"private": true,
"version": "0.0.0",
"type": "module",
"scripts": {
"dev": "vite",
"build": "tsc && vite build",
"preview": "vite preview",
"lint": "eslint --fix --ext .tsx,.ts,.jsx,.js .",
"format": "prettier --write src",
"conflict": "eslint-config-prettier 'src/**/*.{ts,tsx,js,jsx}'",
+ "fix": "tsc && yarn conflict && yarn lint && yarn format"
},
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0"
},
"devDependencies": {
"@types/react": "^18.0.27",
"@types/react-dom": "^18.0.10",
"@typescript-eslint/eslint-plugin": "^5.55.0",
"@typescript-eslint/parser": "^5.55.0",
"@vitejs/plugin-react": "^3.1.0",
"eslint": "^8.36.0",
"eslint-config-prettier": "^8.7.0",
"eslint-plugin-react": "^7.32.2",
"prettier": "^2.8.4",
"typescript": "^4.9.3",
"vite": "^4.1.0",
"vite-plugin-checker": "^0.5.6"
}
}
これで「yarn fix」を行うと以下を行ってくれます。
①typeエラーがないか確認
②eslintとprettierの設定に重複(衝突)するものはないかを確認
③eslintでコードを確認し、修正可能なものは修正する
④prettierでコードをフォーマットする
確認
サーバーの確認
yarn dev
上記のコマンドでviteのサーバーを起動させます。
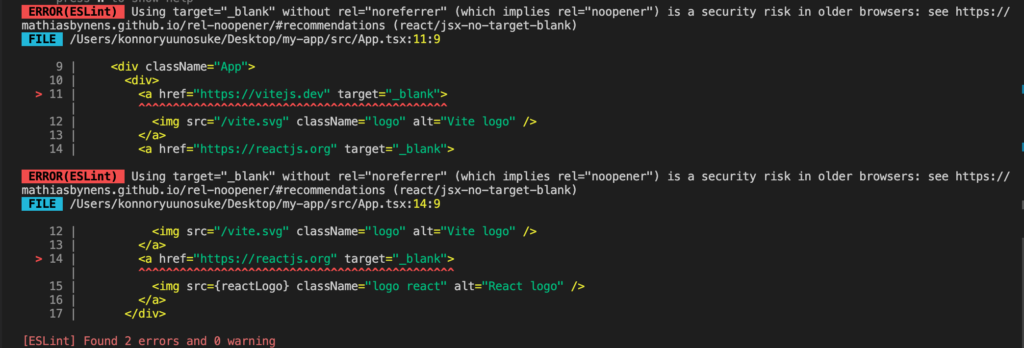
このようにeslintのエラーが表示されれば成功です。
「Ctrl + C」でサーバーを停止させましょう。
コマンドの確認
yarn fix
上記のコマンドで修正コマンドを起動させます。
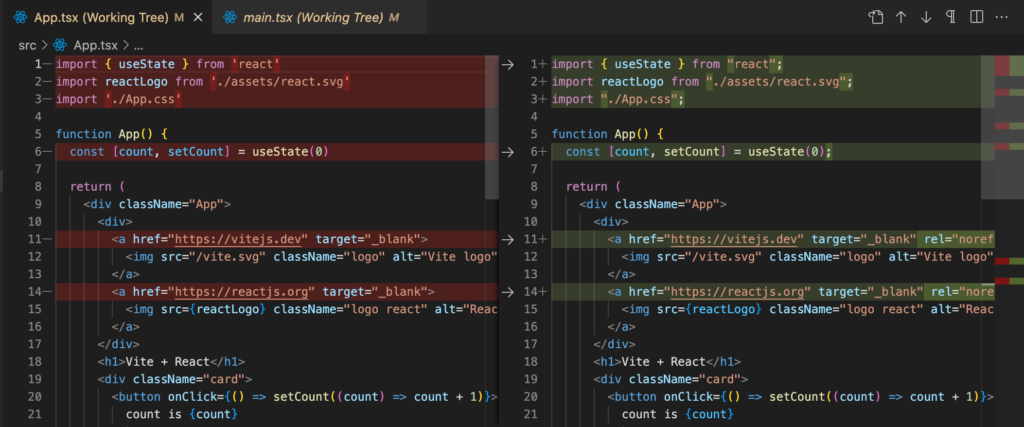
上記のように自動修正がされれば成功です。
おまけ
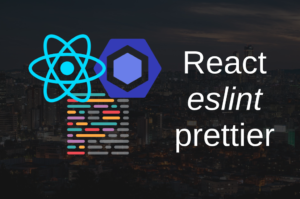
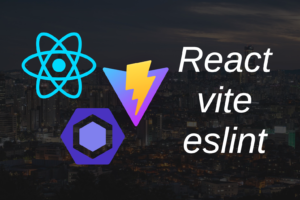