目次
はじめに
今回はViteで作成したReactアプリにEsLintを導入する手順について説明します。
開発サーバーを起動中に、ミスがあればエラーを表示してくれるのでとても便利です。
手順
viteでReactプロジェクトを作成する
yarn create vite my-app --template react
上記のコマンドでviteが搭載されたreactアプリのテンプレートを一発で作成できます。
eslintをインストールと初期設定
yarn add -D eslint
上記のコマンドでeslintのインストールを行います。
yarn eslint --init
上記のコマンドでeslintの初期設定を行います。








いろいろ聞かれるので今回は上記の様に回答します。
{
"env": {
"browser": true,
"es2021": true
},
"extends": [
"eslint:recommended",
"plugin:react/recommended"
],
"overrides": [
],
"parserOptions": {
"ecmaVersion": "latest",
"sourceType": "module"
},
"plugins": [
"react"
],
"rules": {
+ "react/jsx-uses-react": "off",
+ "react/react-in-jsx-scope": "off"
}
}
ルート直下にある「/.eslintrc.json」を上記の様に修正します。
これがないと不要なエラーが出てしまいます。
viteでeslintが起動する様に設定する
yarn add -D vite-plugin-checker
上記のコマンドでvite上でeslintを使う時に必要なライブラリをインストールします。
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
+ import checker from "vite-plugin-checker";
// https://vitejs.dev/config/
export default defineConfig({
plugins: [
react(),
+ checker({
+ typescript: false,
+ eslint: {
+ lintCommand: 'eslint --ext .jsx,.js .',
+ },
+ }),
],
})
上記の様にルート直下にある「vite.config.js」を修正します。
確認
yarn dev
上記のコマンドでvite上でeslintが起動しているか確認しましょう。
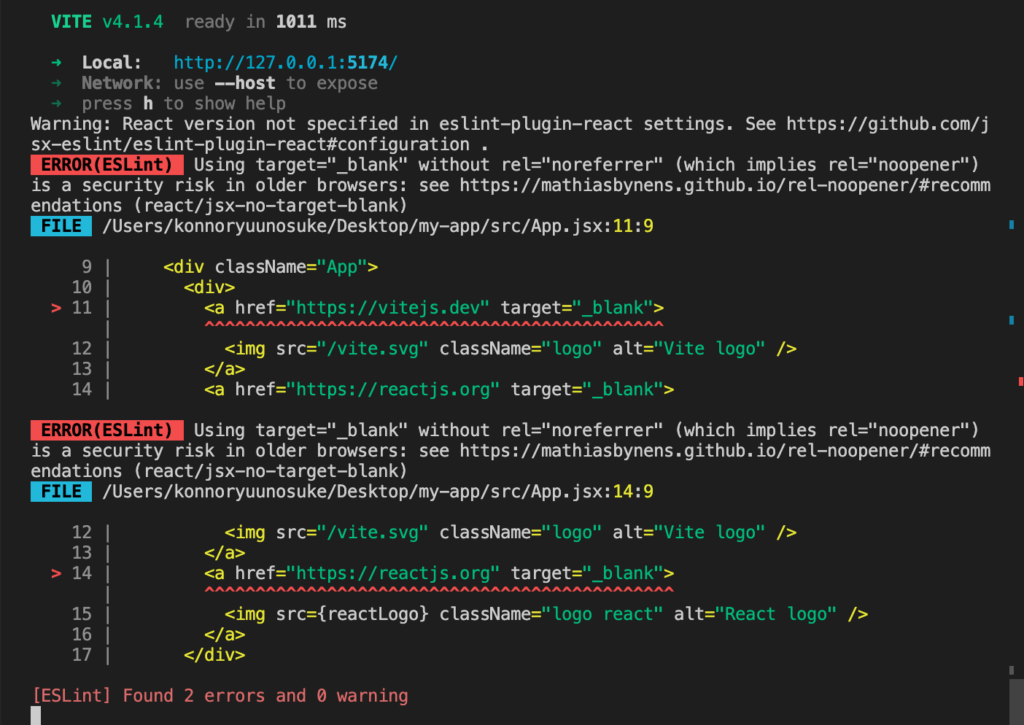
上記の様にeslintのエラーが表示されれば成功です
補足
上記の様に表示されているエラーは下記の様にファイルを修正することで改善されます。
import { useState } from 'react'
import reactLogo from './assets/react.svg'
import './App.css'
function App() {
const [count, setCount] = useState(0)
return (
<div className="App">
<div>
- <a href="https://vitejs.dev" target="_blank">
+ <a href="https://vitejs.dev" target="_blank" rel="noopener noreferrer">
<img src="/vite.svg" className="logo" alt="Vite logo" />
</a>
- <a href="https://reactjs.org" target="_blank">
+ <a href="https://reactjs.org" target="_blank" rel="noopener noreferrer">
<img src={reactLogo} className="logo react" alt="React logo" />
</a>
</div>
<h1>Vite + React</h1>
<div className="card">
<button onClick={() => setCount((count) => count + 1)}>
count is {count}
</button>
<p>
Edit <code>src/App.jsx</code> and save to test HMR
</p>
</div>
<p className="read-the-docs">
Click on the Vite and React logos to learn more
</p>
</div>
)
}
export default App
下記の様に表示されれば成功です。

おまけ
あわせて読みたい

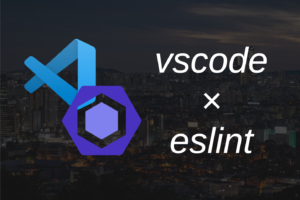
vscodeでファイルを保存時にeslintで自動修正する@React
【はじめに】 今回はvscodeでファイルを編集し保存した時に、自動的にeslintが起動しファイルの修正を行う様に設定します。例ではReactを使用しています。 【手順】 cre...
あわせて読みたい

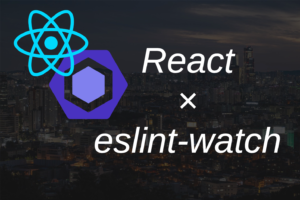
ファイルを保存時にeslint-watchで自動修正する@React
【はじめに】 「ファイルの変更を監視し、自動でeslintを走らせ修正したい。」 今回はeslint-watchというライブラリを使用し、自動でファイルの監視と修正を行います。...