目次
はじめに
こちらは10本の記事で構成されています
- ①Vue.js& Laravel(環境構築)
- ②Vue.js& Laravel(CRUDシステムの準備)
- ③Vue.js& Laravel(Read…データベースからデータを取得)
- ④Vue.js& Laravel(Create…データベースにデータを保存)
- ⑤Vue.js& Laravel(Update…データベースのデータを更新)
- ⑥Vue.js& Laravel(Delete…データベースのデータを削除)
- ⑦Vue.js& Laravel(リアルなダミーデータを大量に挿入)
- ⑧Vue.js& Laravel(検索機能の実装)
- ⑨Vue.js& Laravel(ページャーの実装)
- ⑩Vue.js& Laravel(認証機能の実装)
目標
ページャーを実装する
実装
①Vuetifyのインストール
$ npm install vuetify
$ npm install @mdi/font -D
import VueRouter from 'vue-router';
+ import Vuetify from 'vuetify';
+ import '@mdi/font/css/materialdesignicons.css'
+ import 'vuetify/dist/vuetify.min.css';
require('./bootstrap');
window.Vue = require('vue');
+ Vue.use(Vuetify);
Vue.use(VueRouter);
const router = new VueRouter({
routes: [
{
path: '/task',
name: 'task',
component: () => import('./components/task/Task.vue'),
},
{
path: '/search',
name: 'search',
component: () => import('./components/search/Search.vue'),
},
{
path: '/user',
name: 'user',
component: () => import('./components/user/User.vue'),
},
]
});
Vue.component('app-component', require('./components/AppComponent.vue').default);
const app = new Vue({
el: '#app',
router,
+ vuetify: new Vuetify()
});
これで「①Vuetifyのインストール」が達成されました
②フロントエンドの実装
<template>
<div class="searchComponent">
<form @submit.prevent="taskSearch">
緊急:<input v-model="search.emergency" type="checkbox"><br>
内容:<input v-model="search.content" type="text">
<button>search</button>
</form>
<hr>
+ <div v-show="isShow" class="pagination">
+ <v-pagination v-model="nowPage" :length="maxPages" @input="getNumber"></v-pagination>
+ </div>
<ul>
<li v-for="(task, index) in tasks" :key="index">
緊急:<input type="checkbox" v-model="task.emergency" disabled='disabled'><br>
内容:<span :class="{red:task.emergency}">{{task.content}}</span>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
+ isShow:false,
+ nowPage:1,
+ maxPages:0,
+ itemsNum:1,
+ maxItems:5,
+ taskDatas:[],
search: {
content: "",
emergency: false,
},
tasks:[],
};
},
methods: {
taskSearch(){
axios.post("/api/task/search", this.search).then((res) => {
- this.tasks = res.data;
+ this.taskDatas = res.data;
+ this.getNumber(1);
+ this.isShow = true;
});
},
+ getNumber(page){
+ let maxNum = this.taskDatas.length;
+ this.maxPages = Math.ceil(maxNum / this.maxItems);
+ this.tasks.splice(0, this.tasks.length);
+ for(let i = 0; i < this.maxItems; i++){
+ if(i + this.maxItems * (page - 1) < maxNum -1){
+ this.tasks.push(this.taskDatas[i + this.maxItems * (page - 1)]);
+ }
+ }
+ },
},
};
</script>
<style lang="scss" scoped>
li{
list-style: none;
margin-bottom: 15px;
}
.red{
color: red;
}
+ .pagination{
+ max-width: 500px;
+ margin-bottom: 15px;
+ nav ::v-deep .v-pagination{
+ &__item{
+ color: #000066;
+ border: 1px solid #000066;
+ &--active{
+ color: white;
+ background-color: #000066;
+ }
+ }
+ &__navigation{
+ border: 1px solid #000066;
+ .theme--light.v-icon{
+ color: #000066;
+ }
+ &--disabled{
+ opacity: 0.3;
+ }
+ }
+ }
+ }
</style>
これで「②フロントエンドの実装」が達成されました
次回
あわせて読みたい

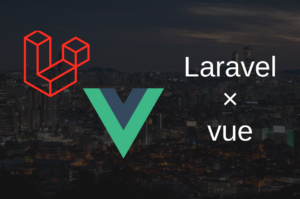
⑩Vue.js & Laravel(認証機能の追加)
【はじめに】 こちらは10本の記事で構成されています ①Vue.js& Laravel(環境構築) ②Vue.js& Laravel(CRUDシステムの準備) ③Vue.js& Laravel(Read...デ...