目次
はじめに
環境
・node -v:v20.12.1
・yarn -v:1.22.19
手順
create-next-appでプロジェクトを作成する
yarn create next-app --typescript my-app
上記のコマンドでプロジェクトを作成しましょう。
いろいろ聞かれますが、下記のように回答します。

jestのインストール
yarn add -D jest jest-environment-jsdom @testing-library/react @testing-library/jest-dom ts-jest @types/jest
上記のコマンドでjestをインストールしましょう。
必要な他のライブラリも同時にインストールしています。
設定を追加する
ルート直下に「jest.config.js」というファイルを作成し、以下のように修正しましょう。
const nextJest = require("next/jest")
/** @type {import('ts-jest').JestConfigWithTsJest} */
/** @type {import("jest").Config} */
const createJestConfig = nextJest({
dir: "./"
})
const customJestConfig = {
preset: "ts-jest",
testEnvironment: "jsdom",
moduleDirectories: ["node_modules", "<rootDir>/"],
moduleNameMapper: {
"@/(.*)$": "<rootDir>/src/$1"
},
testEnvironment: "jest-environment-jsdom"
}
module.exports = createJestConfig(customJestConfig)
コマンドを追加する
{
"name": "my-app",
"version": "0.1.0",
"private": true,
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start",
+ "test": "jest",
"lint": "next lint"
},
"dependencies": {
"next": "14.1.4",
"react": "^18",
"react-dom": "^18"
},
"devDependencies": {
"@testing-library/jest-dom": "^6.4.2",
"@testing-library/react": "^14.2.2",
"@types/jest": "^29.5.12",
"@types/node": "^20",
"@types/react": "^18",
"@types/react-dom": "^18",
"eslint": "^8",
"eslint-config-next": "14.1.4",
"jest": "^29.7.0",
"jest-environment-jsdom": "^29.7.0",
"ts-jest": "^29.1.2",
"typescript": "^5"
}
}
これで「yarn test」でjestが動くようになりました
テストファイルを作成
「src/pages/index.test.tsx」を作成し、下記のように記述します。
import "@testing-library/jest-dom";
import Home from "./";
import { render } from "@testing-library/react";
describe("Home", () => {
test("「Hello Next」という文字が存在する", async () => {
const { getByText } = render(<Home />);
expect(getByText("Hello Next")).toBeInTheDocument();
});
});
「Hello Next」という文字が存在することを確認するテストコードです。
エラーを表示させてみる
yarn test
上記のコマンドでjestを使用してみましょう。
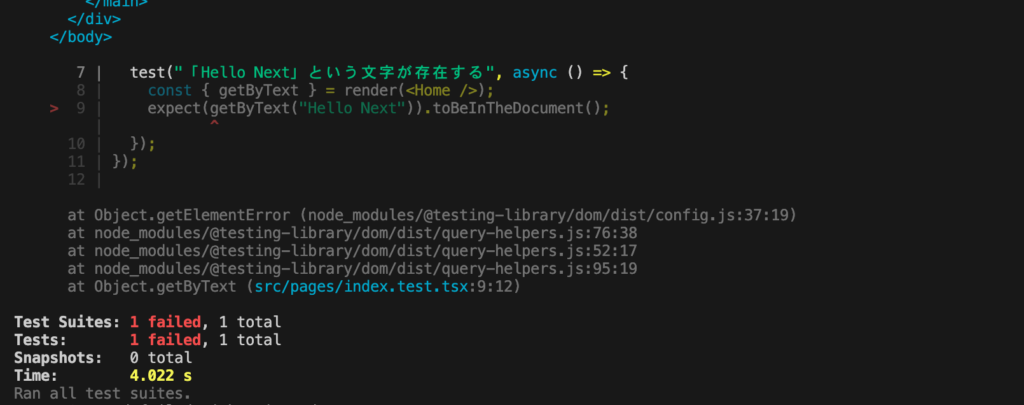
対象の「<Home/>」コンポーネントに「Hello Next」という文字が存在しないのでエラーが起きます。
エラーを解決する
「src/pages/index.tsx」を下記の様に修正します。
import Head from "next/head";
import Image from "next/image";
import { Inter } from "next/font/google";
import styles from "@/styles/Home.module.css";
const inter = Inter({ subsets: ["latin"] });
export default function Home() {
return (
<>
<Head>
<title>Create Next App</title>
<meta name="description" content="Generated by create next app" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link rel="icon" href="/favicon.ico" />
</Head>
<main className={`${styles.main} ${inter.className}`}>
+ <p>Hello Next</p>
<div className={styles.description}>
<p>
Get started by editing
<code className={styles.code}>src/pages/index.tsx</code>
</p>
<div>
<a
href="https://vercel.com?utm_source=create-next-app&utm_medium=default-template&utm_campaign=create-next-app"
target="_blank"
rel="noopener noreferrer"
>
By{" "}
<Image
src="/vercel.svg"
alt="Vercel Logo"
className={styles.vercelLogo}
width={100}
height={24}
priority
/>
</a>
</div>
</div>
<div className={styles.center}>
<Image
className={styles.logo}
src="/next.svg"
alt="Next.js Logo"
width={180}
height={37}
priority
/>
</div>
<div className={styles.grid}>
<a
href="https://nextjs.org/docs?utm_source=create-next-app&utm_medium=default-template&utm_campaign=create-next-app"
className={styles.card}
target="_blank"
rel="noopener noreferrer"
>
<h2>
Docs <span>-></span>
</h2>
<p>
Find in-depth information about Next.js features and API.
</p>
</a>
<a
href="https://nextjs.org/learn?utm_source=create-next-app&utm_medium=default-template&utm_campaign=create-next-app"
className={styles.card}
target="_blank"
rel="noopener noreferrer"
>
<h2>
Learn <span>-></span>
</h2>
<p>
Learn about Next.js in an interactive course with quizzes!
</p>
</a>
<a
href="https://vercel.com/templates?framework=next.js&utm_source=create-next-app&utm_medium=default-template&utm_campaign=create-next-app"
className={styles.card}
target="_blank"
rel="noopener noreferrer"
>
<h2>
Templates <span>-></span>
</h2>
<p>
Discover and deploy boilerplate example Next.js projects.
</p>
</a>
<a
href="https://vercel.com/new?utm_source=create-next-app&utm_medium=default-template&utm_campaign=create-next-app"
className={styles.card}
target="_blank"
rel="noopener noreferrer"
>
<h2>
Deploy <span>-></span>
</h2>
<p>
Instantly deploy your Next.js site to a shareable URL
with Vercel.
</p>
</a>
</div>
</main>
</>
);
}
これで「<Home />」コンポーネント内に「Hello Next」という文字が存在するのでエラーが解決されるはずです。
yarn test
上記のコマンドを叩き下記の様にエラーが解決されていれば成功です。
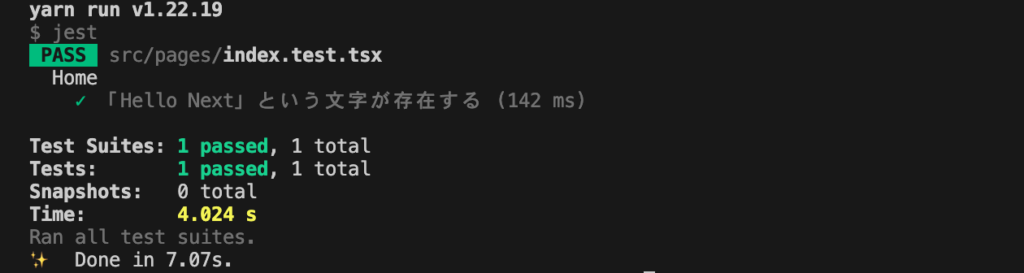
あわせて読みたい

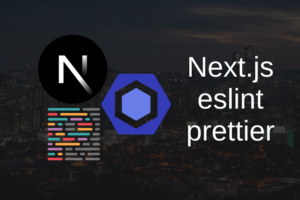
Next.jsでeslintとprettierを使用する
【はじめに】 環境 ・node -v:v20.12.1・yarn -v:1.22.19 create-next-appでプロジェクトを作成する yarn create next-app --typescript my-app 上記のコマンドでプロ...